How to access server MBean properties at weblogic 11g using Java – JMX
If you want to get any property of Admin or Managed Server (Weblogic) using Java, then this post will help you.
The Oracle Enterprise Manager have a useful tool for explore MBean (System MBean Browser).
As you can see in the picture bellow:
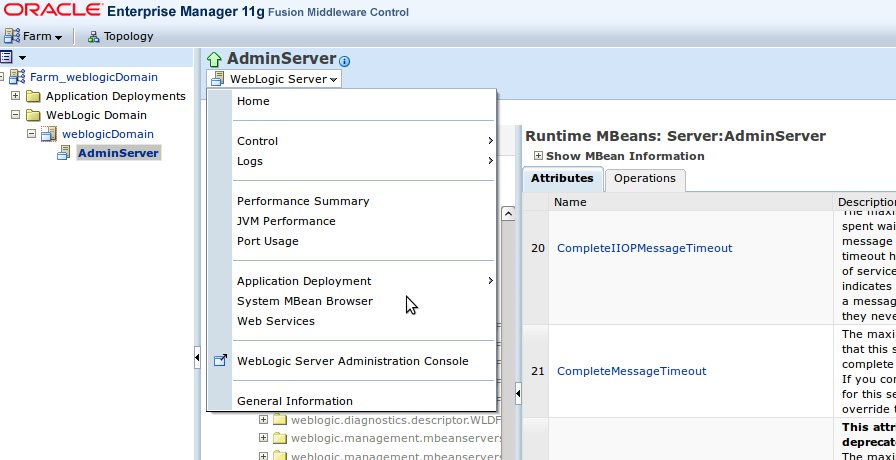
Do you can to capture any server property desired with these java code:
1 – Reading a property if the application is deployed at server (local connection)
import javax.management.MBeanServer; import javax.management.ObjectName; import javax.naming.InitialContext; String serverName = System.getProperty("weblogic.Name"); InitialContext ctx = new InitialContext(); MBeanServer server = (MBeanServer)ctx.lookup("java:comp/env/jmx/runtime"); ObjectName objName = new ObjectName("com.bea:Name=" + serverName + ",Type=Server"); String pathJKS = (String) server.getAttribute(objName, "CustomTrustKeyStoreFileName");
2 – Reading a property if the application is remote (remote connection)
import java.util.Hashtable; import javax.management.ObjectName; import javax.management.remote.JMXConnector; import javax.management.remote.JMXConnectorFactory; import javax.management.remote.JMXServiceURL; import javax.naming.Context; public static void main(String [] args) throws Exception{ String serverName = "AdminServer"; String hostName = "192.168.1.192"; String username = "weblogic"; String password = "welcome1"; int port = 10000; String protocol = "t3"; String jndiroot = "/jndi/"; String mserver = "weblogic.management.mbeanservers.domainruntime"; JMXServiceURL serviceURL = new JMXServiceURL(protocol, hostName, port, jndiroot + mserver); Hashtable h = new Hashtable(); h.put(Context.SECURITY_PRINCIPAL, username); h.put(Context.SECURITY_CREDENTIALS, password); h.put(JMXConnectorFactory.PROTOCOL_PROVIDER_PACKAGES, "weblogic.management.remote"); JMXConnector connector = JMXConnectorFactory.connect(serviceURL, h); ObjectName objName = new ObjectName("com.bea:Name=" + serverName + ",Type=Server"); String pathJKS = (String) connector.getMBeanServerConnection().getAttribute(objName, "CustomTrustKeyStoreFileName"); System.out.println(pathJKS); }
In the cases above, i’m reading a property that is called “CustomTrustKeyStoreFileName”, that’s a string path of the Trusted Keystore. But many and many properties could be read, for example:
- CustomIdentityKeyStoreFileName
- ListenPort
- UploadDirectoryName
That’s it.
Victor Jabur